Monday, April 20, 2015
Friday, April 17, 2015
Creating View and Controller
this is the second article continue with angular basics and followed with the http://cgenit.blogspot.com/2015/04/angular.html
Here we are going to discuss the first major concept, View and controller.
Angular provides a nice mechanism called view and controller which enhances the readability and re-usability of the code.
controller has the data and the function. controller bind to view with something called $scope.
This is the glue between controller and the view.
Controller need not to know anything about the View and View does not want to know anything about the Controller. This achieved simply by using the concept of $scope.
angular has lots of objects defined with the $ sign and you will see them as you dig up more with the angular.
Let's go to example, let's try to do the same example we did to customer data set using Controller.
---------------------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-controller="SimpleController">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li
data-ng-repeat="customer in customers | filter:name | orderBy:'city'">{{customer.name
| uppercase}}- {{customer.city | lowercase}}</li>
</ul>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('SimpleController', function($scope) {
$scope.customers = [ {
name : 'Shaun',
city : 'Phoenix'
}, {
name : 'Dilan',
city : 'Chicago'
}, {
name : 'Mike',
city : 'NewYork'
} ];
});
</script>
</body>
</html>
-------------------------------------------------------------------------------------------------------------------
We haven't change the logic used in previous example in http://cgenit.blogspot.com/2015/04/angular.html
Here we have introduced a Controller.
Before that we have given a name to the application "myApp". If you see the opening HTML tag you will that.
Then we move to definition of the Controller.
We have defined inside separate area , as a separate script. If you familiar with packaging and modularising , now you should see that.
We are defining the Controller in a separate script, inside "script" tags.
First, we define a variable or a holder for application.
var app = angular.module('myApp', []);
we have defined a variable "app" or module with module giving our application name "myApp"
don't worry about empty braces.. well that's for dependency injection.. or we can say module myApp depend on applications, can be given inside those.
Second , then we define the Controller
we define the controller as function. this is more like simple java-script function. we have included $scope in the Controller definition. This is scope which acts as a glue between Controller and the View.
As we have included Controller inside a separate script , you can simply include that into separate file and include in the main file.
So when we are using Controller , with use of data-ng-controller , we give the name of the Controller we are using. the functions and the data defined within the scope of the specified Controller will be available. In this case , we have given inside a div tag, and "SimpleController" Controller data and functions will be available within the div tag.
So when you run the above code , you will see the same output as the previous example.
Here we are going to discuss the first major concept, View and controller.
Angular provides a nice mechanism called view and controller which enhances the readability and re-usability of the code.
controller has the data and the function. controller bind to view with something called $scope.
This is the glue between controller and the view.
Controller need not to know anything about the View and View does not want to know anything about the Controller. This achieved simply by using the concept of $scope.
angular has lots of objects defined with the $ sign and you will see them as you dig up more with the angular.
Let's go to example, let's try to do the same example we did to customer data set using Controller.
---------------------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="myApp">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-controller="SimpleController">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li
data-ng-repeat="customer in customers | filter:name | orderBy:'city'">{{customer.name
| uppercase}}- {{customer.city | lowercase}}</li>
</ul>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('SimpleController', function($scope) {
$scope.customers = [ {
name : 'Shaun',
city : 'Phoenix'
}, {
name : 'Dilan',
city : 'Chicago'
}, {
name : 'Mike',
city : 'NewYork'
} ];
});
</script>
</body>
</html>
-------------------------------------------------------------------------------------------------------------------
We haven't change the logic used in previous example in http://cgenit.blogspot.com/2015/04/angular.html
Here we have introduced a Controller.
Before that we have given a name to the application "myApp". If you see the opening HTML tag you will that.
Then we move to definition of the Controller.
We have defined inside separate area , as a separate script. If you familiar with packaging and modularising , now you should see that.
We are defining the Controller in a separate script, inside "script" tags.
First, we define a variable or a holder for application.
var app = angular.module('myApp', []);
we have defined a variable "app" or module with module giving our application name "myApp"
don't worry about empty braces.. well that's for dependency injection.. or we can say module myApp depend on applications, can be given inside those.
Second , then we define the Controller
we define the controller as function. this is more like simple java-script function. we have included $scope in the Controller definition. This is scope which acts as a glue between Controller and the View.
As we have included Controller inside a separate script , you can simply include that into separate file and include in the main file.
So when we are using Controller , with use of data-ng-controller , we give the name of the Controller we are using. the functions and the data defined within the scope of the specified Controller will be available. In this case , we have given inside a div tag, and "SimpleController" Controller data and functions will be available within the div tag.
So when you run the above code , you will see the same output as the previous example.
Angular
AngularJs, commonly called as "Angular" is an open source web application framework mainly maintained by Google and community of developers, which helps a lot in development of SPA, Single Page Application.
You can download Angular from
https://angularjs.org/
and for more information
http://en.wikipedia.org/wiki/AngularJS
http://www.w3schools.com/angular/
Here i am going to discuss few key words associated with Angular. Those include
Why Angular ??? It's framework... Good SPA framework
SPA ???? Single Page Application
As a framework it gives
Data Binding, MVC, Routing , Testing, jqLite, Templates, History, Factories etc.
jqLite - for DOM manipulation.
So it has
ViewModel , Controllers , Views , Directives , Services , Dependency Injection , Validation much more..
First we create simple html page and add the angular to it.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
</body>
</html>
----------------------------------------------------------------------------------------------------
I have added the reference to the web, you can download the angular from site https://angularjs.org/ and give the reference straight.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="angular.min.js"></script>
</head>
<body>
</body>
</html>
----------------------------------------------------------------------------------------------------
Now we are ready to go with the Angular, So once we add the Angular to page.. Next we will look at key concepts... Directive , Data Binding ...
Directive
Angular extend HTML with ng-directive. So directive start with ng-
angular has various directives and some are
ng-app = directive defines AngularJs application
ng-model = directive binds the value of the HTML control (input, slect, textarea..) to application data
ng-bind = directive binds application data to the HTML view
Now let's write a simple angular application with directives
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
<p ng-bind="name"></p>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
You can see above, highlighted, i have used few directives.
ng-app - declares this as an angular application
then we have we have input text box and we have bind the value of the text box to application using ng-model directive , we have use "name" as the reference.
Now we need to show the application data back into HTML view.
ng-bind directive binds that application data to the HTML view.
That's how angular application is defined and it's data been bind into application and show application bound data back into HTML view.
Behind the scene : What ng-model does behind the scene is, it's going to add a property up in the memory called 'name' into what's called the "scope". you will understand this concept, as you will read through.
Now we have learnt three basic angular directives.
Note : without using ng-bind , we can use {{}} to bind application data to HTML view.
----------------------------------------------------------------------------------------------------
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
{{name}}
</div>
----------------------------------------------------------------------------------------------------
This will have the same results
{{name}} , this is known as data binding expression.
So we now know expressions also, this is much similar to JavaScript expressions..
Now we have learnt four key words, Next ..
let's do the iteration.. key feature in any language..
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div >
<p>Name: <input type="text" data-ng-model="name"></p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
As you can see we have defines a new angular application.
Previously we have defined ng-app directive inside the div tag
so if we need to define a new application then we need to define ng-app in the next div tag also.
to avoid that we have added the ng-app directive to the html root element.
by doing, we have defined the whole app as a angular application.
second div tag contains the repeated elements.
here we have defined an array of elements. to do that we have used another angular directive,
data-ng-init , this directive initializes the names array in to the application.
Now we have initialized the elements of array.
let's repeat the elements and show that inside the HTML view.
to that we have used data-ng-repeat directive, this helps us to define and loop or iterate over the elements. we are now iterating over the elements in the names array.
we have defined the repeat element with "personName in names"
Here "personName" is the temp[orary holder while "names" is the array of elements we are using to repeat. This is much similar to foreach loop we are using in javascipt or Java.
Then we bind the application data inside the iteration, or the repeat element with data binding expression {{personName}}
Note : we have used the temporary value holder to bind data to the HTML view from the angular application.
This will have output as below, above the "Looping with the ng-repeat Directive" will have the text box of previous application. you can see the output by saving the code into notepad and save as html file and running ina browser.
output
===========================================================
So we have learnt few directives.
go to https://docs.angularjs.org/api
you will have all the directive list with their documentation.
Now let's look at filters
To discuss filters we define new set of data elements as Customers and do the filtering for properties of customer.
we can use the ng-init to initialize the customers data.
First we initialize data and print that.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="customer in customers">{{customer.name}}- {{customer.city}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
This will print the name and city pairs of customers.
Now let's add the filter.
We have the name text filed , which we had added in the first application. this is third application.
let's use name as a filter.
----------------------------------------------------------------------------------------------------
----------------------------------------------------------------------------------------------------
it's very simple.
Just add the pipe character and filter word as shown in highlighted text above.
After the filter, give the property you are using to filter. Here we have used "name", that is data reference used to bind html text box data to application.
you can see the dynamically list will change in the HTML view.
very smooth and simple.
we can give order by filter also.
let's put order by to city
----------------------------------------------------------------------------------------------------
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="customer in customers | filter:name | orderBy : 'city'">{{customer.name}}- {{customer.city}}</li>
</ul>
</div>
----------------------------------------------------------------------------------------------------
Note : we have given order by field property value inside single quotes.
This will arrange data order by city name.
we can use more filters.
let's add uppercase to names and lowercase to city values.
the whole code will be as follows
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li
data-ng-repeat="customer in customers | filter:name | orderBy:'city'">{{customer.name
| uppercase}}- {{customer.city | lowercase}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
you can define your own custom filters. to look at all the filters provided by the angular go to
https://docs.angularjs.org/api and goto filters section.
https://docs.angularjs.org/api/ng/filter
you will see the list of filters provided by the angular.
You can download Angular from
https://angularjs.org/
and for more information
http://en.wikipedia.org/wiki/AngularJS
http://www.w3schools.com/angular/
Here i am going to discuss few key words associated with Angular. Those include
- Directives, Filters, and Data Binding , Expressions
- Views, Controllers and Scope
- Modules, Routers and Factories
Why Angular ??? It's framework... Good SPA framework
SPA ???? Single Page Application
As a framework it gives
Data Binding, MVC, Routing , Testing, jqLite, Templates, History, Factories etc.
jqLite - for DOM manipulation.
So it has
ViewModel , Controllers , Views , Directives , Services , Dependency Injection , Validation much more..
First we create simple html page and add the angular to it.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
</body>
</html>
----------------------------------------------------------------------------------------------------
I have added the reference to the web, you can download the angular from site https://angularjs.org/ and give the reference straight.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="angular.min.js"></script>
</head>
<body>
</body>
</html>
----------------------------------------------------------------------------------------------------
Now we are ready to go with the Angular, So once we add the Angular to page.. Next we will look at key concepts... Directive , Data Binding ...
Directive
Angular extend HTML with ng-directive. So directive start with ng-
angular has various directives and some are
ng-app = directive defines AngularJs application
ng-model = directive binds the value of the HTML control (input, slect, textarea..) to application data
ng-bind = directive binds application data to the HTML view
Now let's write a simple angular application with directives
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
<p ng-bind="name"></p>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
You can see above, highlighted, i have used few directives.
ng-app - declares this as an angular application
then we have we have input text box and we have bind the value of the text box to application using ng-model directive , we have use "name" as the reference.
Now we need to show the application data back into HTML view.
ng-bind directive binds that application data to the HTML view.
That's how angular application is defined and it's data been bind into application and show application bound data back into HTML view.
Behind the scene : What ng-model does behind the scene is, it's going to add a property up in the memory called 'name' into what's called the "scope". you will understand this concept, as you will read through.
Now we have learnt three basic angular directives.
Note : without using ng-bind , we can use {{}} to bind application data to HTML view.
----------------------------------------------------------------------------------------------------
<div ng-app="">
<p>Name: <input type="text" ng-model="name"></p>
{{name}}
</div>
----------------------------------------------------------------------------------------------------
This will have the same results
{{name}} , this is known as data binding expression.
So we now know expressions also, this is much similar to JavaScript expressions..
Now we have learnt four key words, Next ..
let's do the iteration.. key feature in any language..
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div >
<p>Name: <input type="text" data-ng-model="name"></p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
As you can see we have defines a new angular application.
Previously we have defined ng-app directive inside the div tag
so if we need to define a new application then we need to define ng-app in the next div tag also.
to avoid that we have added the ng-app directive to the html root element.
by doing, we have defined the whole app as a angular application.
second div tag contains the repeated elements.
here we have defined an array of elements. to do that we have used another angular directive,
data-ng-init , this directive initializes the names array in to the application.
Now we have initialized the elements of array.
let's repeat the elements and show that inside the HTML view.
to that we have used data-ng-repeat directive, this helps us to define and loop or iterate over the elements. we are now iterating over the elements in the names array.
we have defined the repeat element with "personName in names"
Here "personName" is the temp[orary holder while "names" is the array of elements we are using to repeat. This is much similar to foreach loop we are using in javascipt or Java.
Then we bind the application data inside the iteration, or the repeat element with data binding expression {{personName}}
Note : we have used the temporary value holder to bind data to the HTML view from the angular application.
This will have output as below, above the "Looping with the ng-repeat Directive" will have the text box of previous application. you can see the output by saving the code into notepad and save as html file and running ina browser.
output
===========================================================
Looping with the ng-repeat Directive
- Shaun
- Dilan
- Mike
- Paul
So we have learnt few directives.
go to https://docs.angularjs.org/api
you will have all the directive list with their documentation.
Now let's look at filters
To discuss filters we define new set of data elements as Customers and do the filtering for properties of customer.
we can use the ng-init to initialize the customers data.
First we initialize data and print that.
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="customer in customers">{{customer.name}}- {{customer.city}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
This will print the name and city pairs of customers.
Now let's add the filter.
We have the name text filed , which we had added in the first application. this is third application.
let's use name as a filter.
----------------------------------------------------------------------------------------------------
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
{{customer.name}}- {{customer.city}}
Looping with the ng-repeat Directive
----------------------------------------------------------------------------------------------------
it's very simple.
Just add the pipe character and filter word as shown in highlighted text above.
After the filter, give the property you are using to filter. Here we have used "name", that is data reference used to bind html text box data to application.
you can see the dynamically list will change in the HTML view.
very smooth and simple.
we can give order by filter also.
let's put order by to city
----------------------------------------------------------------------------------------------------
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="customer in customers | filter:name | orderBy : 'city'">{{customer.name}}- {{customer.city}}</li>
</ul>
</div>
----------------------------------------------------------------------------------------------------
Note : we have given order by field property value inside single quotes.
This will arrange data order by city name.
we can use more filters.
let's add uppercase to names and lowercase to city values.
the whole code will be as follows
----------------------------------------------------------------------------------------------------
<!DOCTYPE html>
<html data-ng-app="">
<head>
<meta charset="ISO-8859-1">
<title>Insert title here</title>
<script
src="http://ajax.googleapis.com/ajax/libs/angularjs/1.3.14/angular.min.js"></script>
</head>
<body>
<div>
<p>
Name: <input type="text" data-ng-model="name">
</p>
{{name}}
</div>
<div data-ng-init="names=['Shaun','Dilan', 'Mike', 'Paul']">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li data-ng-repeat="personName in names">{{personName}}</li>
</ul>
</div>
<div
data-ng-init="customers=[{name:'Shaun',city:'Phoenix'},{name:'Dilan',city:'Chicago'},{name:'Mike',city:'NewYork'}]">
<h3>Looping with the ng-repeat Directive</h3>
<ul>
<li
data-ng-repeat="customer in customers | filter:name | orderBy:'city'">{{customer.name
| uppercase}}- {{customer.city | lowercase}}</li>
</ul>
</div>
</body>
</html>
----------------------------------------------------------------------------------------------------
you can define your own custom filters. to look at all the filters provided by the angular go to
https://docs.angularjs.org/api and goto filters section.
https://docs.angularjs.org/api/ng/filter
you will see the list of filters provided by the angular.
Thursday, April 16, 2015
Spring Expression Language
http://docs.spring.io/spring/docs/current/spring-framework-reference/html/expressions.html
You can find very descriptive details from the above mention link in the spring docs. The definition include as below..
The Spring Expression Language (SpEL for short) is a powerful expression language that supports querying and manipulating an object graph at runtime. The language syntax is similar to Unified EL but offers additional features, most notably method invocation and basic string templating functionality.
I am not going to rewrite what has already being well described with the spring docs, instead i thought of sharing some exceptions which might you experience.
This is about the initial example of "Hello world " as Spring expression.
You can simply create a class and inside main method you can directly run the code. I have just included inside static method.
So below is the code
ExpressionEvaluationWithSEI.java
------------------------------------------------------------------------------------------------------
package com.spel;
import org.springframework.expression.Expression;
import org.springframework.expression.ExpressionParser;
import org.springframework.expression.spel.standard.SpelExpressionParser;
/**
* http://docs.spring.io/spring/docs/current/spring-framework-reference/html/
* expressions.html
*
* Expression Evaluation using Spring’s Expression Interface
*
* @author APrasad
*
*/
public class ExpressionEvaluationWithSEI {
public static void evaluateLiteralStringExpression() {
ExpressionParser parser = new SpelExpressionParser();
Expression expression = parser.parseExpression("'Hello World'");
String message = (String)expression.getValue();
System.out.println("message ="+message);
}
public static void main(String[] args) {
ExpressionEvaluationWithSEI.evaluateLiteralStringExpression();
}
}
------------------------------------------------------------------------------------------------------
This will have simple output
output
=============================================================
message =Hello World
=============================================================
Note : The word "Hello World" is within the single quotes..... So if any case if you miss the single quotes , then you will probably witness below exception.
change new code
------------------------------------------------------------------------------------------------------
Expression expression = parser.parseExpression("Hello World");
//Note :: I have remove the single quotes around the literal expression Hello World
------------------------------------------------------------------------------------------------------
output
=============================================================
Exception in thread "main" org.springframework.expression.spel.SpelParseException: EL1041E:(pos 6): After parsing a valid expression, there is still more data in the expression: 'World'
at org.springframework.expression.spel.standard.InternalSpelExpressionParser.doParseExpression(InternalSpelExpressionParser.java:118)
at org.springframework.expression.spel.standard.SpelExpressionParser.doParseExpression(SpelExpressionParser.java:56)
at org.springframework.expression.spel.standard.SpelExpressionParser.doParseExpression(SpelExpressionParser.java:1)
at org.springframework.expression.common.TemplateAwareExpressionParser.parseExpression(TemplateAwareExpressionParser.java:66)
at org.springframework.expression.common.TemplateAwareExpressionParser.parseExpression(TemplateAwareExpressionParser.java:56)
at com.spel.ExpressionEvaluationWithSEI.evaluateLiteralStringExpression(ExpressionEvaluationWithSEI.java:22)
at com.spel.ExpressionEvaluationWithSEI.main(ExpressionEvaluationWithSEI.java:29)
=============================================================
Now let's also check other functionality given with the expression.
ExpressionEvaluationWithSEI.java
------------------------------------------------------------------------------------------------------
package com.spel;
import org.springframework.expression.Expression;
import org.springframework.expression.ExpressionParser;
import org.springframework.expression.spel.standard.SpelExpressionParser;
/**
* http://docs.spring.io/spring/docs/current/spring-framework-reference/html/
* expressions.html
*
* Expression Evaluation using Spring’s Expression Interface
*
* @author APrasad
*
*/
public class ExpressionEvaluationWithSEI {
public static void evaluateLiteralStringExpression() {
ExpressionParser parser = new SpelExpressionParser();
Expression expression = parser.parseExpression("'Hello World'");
String message = (String)expression.getValue();
System.out.println("message ="+message);
//newly added
System.out.println("getExpressionString ="+expression.getExpressionString());
System.out.println("toString ="+expression.toString());
System.out.println("getValueType ="+expression.getValueType());
}
public static void main(String[] args) {
ExpressionEvaluationWithSEI.evaluateLiteralStringExpression();
}
}
------------------------------------------------------------------------------------------------------
output
=============================================================
message =Hello World
getExpressionString ='Hello World'
toString =org.springframework.expression.spel.standard.SpelExpression@18019707
getValueType =class java.lang.String
=============================================================
You can find very descriptive details from the above mention link in the spring docs. The definition include as below..
The Spring Expression Language (SpEL for short) is a powerful expression language that supports querying and manipulating an object graph at runtime. The language syntax is similar to Unified EL but offers additional features, most notably method invocation and basic string templating functionality.
I am not going to rewrite what has already being well described with the spring docs, instead i thought of sharing some exceptions which might you experience.
This is about the initial example of "Hello world " as Spring expression.
You can simply create a class and inside main method you can directly run the code. I have just included inside static method.
So below is the code
ExpressionEvaluationWithSEI.java
------------------------------------------------------------------------------------------------------
package com.spel;
import org.springframework.expression.Expression;
import org.springframework.expression.ExpressionParser;
import org.springframework.expression.spel.standard.SpelExpressionParser;
/**
* http://docs.spring.io/spring/docs/current/spring-framework-reference/html/
* expressions.html
*
* Expression Evaluation using Spring’s Expression Interface
*
* @author APrasad
*
*/
public class ExpressionEvaluationWithSEI {
public static void evaluateLiteralStringExpression() {
ExpressionParser parser = new SpelExpressionParser();
Expression expression = parser.parseExpression("'Hello World'");
String message = (String)expression.getValue();
System.out.println("message ="+message);
}
public static void main(String[] args) {
ExpressionEvaluationWithSEI.evaluateLiteralStringExpression();
}
}
------------------------------------------------------------------------------------------------------
This will have simple output
output
=============================================================
message =Hello World
=============================================================
Note : The word "Hello World" is within the single quotes..... So if any case if you miss the single quotes , then you will probably witness below exception.
change new code
------------------------------------------------------------------------------------------------------
Expression expression = parser.parseExpression("Hello World");
//Note :: I have remove the single quotes around the literal expression Hello World
------------------------------------------------------------------------------------------------------
output
=============================================================
Exception in thread "main" org.springframework.expression.spel.SpelParseException: EL1041E:(pos 6): After parsing a valid expression, there is still more data in the expression: 'World'
at org.springframework.expression.spel.standard.InternalSpelExpressionParser.doParseExpression(InternalSpelExpressionParser.java:118)
at org.springframework.expression.spel.standard.SpelExpressionParser.doParseExpression(SpelExpressionParser.java:56)
at org.springframework.expression.spel.standard.SpelExpressionParser.doParseExpression(SpelExpressionParser.java:1)
at org.springframework.expression.common.TemplateAwareExpressionParser.parseExpression(TemplateAwareExpressionParser.java:66)
at org.springframework.expression.common.TemplateAwareExpressionParser.parseExpression(TemplateAwareExpressionParser.java:56)
at com.spel.ExpressionEvaluationWithSEI.evaluateLiteralStringExpression(ExpressionEvaluationWithSEI.java:22)
at com.spel.ExpressionEvaluationWithSEI.main(ExpressionEvaluationWithSEI.java:29)
Now let's also check other functionality given with the expression.
ExpressionEvaluationWithSEI.java
------------------------------------------------------------------------------------------------------
package com.spel;
import org.springframework.expression.Expression;
import org.springframework.expression.ExpressionParser;
import org.springframework.expression.spel.standard.SpelExpressionParser;
/**
* http://docs.spring.io/spring/docs/current/spring-framework-reference/html/
* expressions.html
*
* Expression Evaluation using Spring’s Expression Interface
*
* @author APrasad
*
*/
public class ExpressionEvaluationWithSEI {
public static void evaluateLiteralStringExpression() {
ExpressionParser parser = new SpelExpressionParser();
Expression expression = parser.parseExpression("'Hello World'");
String message = (String)expression.getValue();
System.out.println("message ="+message);
//newly added
System.out.println("getExpressionString ="+expression.getExpressionString());
System.out.println("toString ="+expression.toString());
System.out.println("getValueType ="+expression.getValueType());
}
public static void main(String[] args) {
ExpressionEvaluationWithSEI.evaluateLiteralStringExpression();
}
}
output
=============================================================
message =Hello World
getExpressionString ='Hello World'
toString =org.springframework.expression.spel.standard.SpelExpression@18019707
getValueType =class java.lang.String
Friday, April 10, 2015
Tricky Codes used to test your knowledge on Java
I have came a cross few tricky Java coding. It's not difficult stuff, but pretty much tricky, where anybody will easily miss.
1.
package array;
public class ChangeIt {
static void doIt(int[] z) {
z = null;
}
}
2. Above ChangeIt class sets the for "null", let's look at copying to separate array.
package array;
public class ChangeIt {
static void doIt(int[] z){
int A[] =z;
A[0] =99;
}
}
package array;
public class TestIt {
public static void main(String[] args) {
int[] myArray = { 1, 2, 3, 4, 5 };
ChangeIt.doIt(myArray);
for (int i = 0; i < myArray.length; i++) {
System.out.print(myArray[i] + " ");
}
}
}
output
==============================
99 2 3 4 5
==============================
1.
package array;
public class ChangeIt {
static void doIt(int[] z) {
z = null;
}
}
package array;
public class TestIt {
public static void main(String[] args) {
int[] myArray = { 1, 2, 3, 4, 5 };
ChangeIt.doIt(myArray);
for (int i = 0; i < myArray.length; i++) {
System.out.print(myArray[i] + " ");
}
}
}
Output :::
----------------
1 2 3 4 5
inside method "ChangeIt.doIt(myArray);" array reference is set to null. But it will be a copy of the object , not the reference.
But if you try to loop array inside method, then there will be NullPointerException
eg : I have highlighted the code which will make NullPointerException.
package array;
public class ChangeIt {
static void doIt(int[] z) {
z = null;
for (int i = 0; i < z.length; i++) {
System.out.print(z[i] + " ");
}
}
}
Output
------------
Exception in thread "main" java.lang.NullPointerException
at array.ChangeIt.doIt(ChangeIt.java:8)
at array.TestIt.main(TestIt.java:8)
---------------
2. Above ChangeIt class sets the for "null", let's look at copying to separate array.
package array;
public class ChangeIt {
static void doIt(int[] z){
int A[] =z;
A[0] =99;
}
}
package array;
public class TestIt {
public static void main(String[] args) {
int[] myArray = { 1, 2, 3, 4, 5 };
ChangeIt.doIt(myArray);
for (int i = 0; i < myArray.length; i++) {
System.out.print(myArray[i] + " ");
}
}
}
output
==============================
99 2 3 4 5
==============================
Even though we are changing the value of newly create array, it still has the reference to passes array (in this case "myArray" or the array comes from the parameter of that method). you may miss that it still has the reference and give output something like "1 2 3 4 5 " which is wrong.
3. Using "this" inside static context - this will not allowed inside static context.
This will have compile error.
----------------------------------------------------------------------
public static void main(String[] args) {
System.out.println(this);
}
----------------------------------------------------------------------
But you can use "this" out of static context.
-----------------------------------------------------------------------
public class Circle {
private String name;
public String getName() {
// this is allowed
System.out.println(this);
return name;
}
public void setName(String name) {
this.name = name;
}
}
---------------------------------------------------------------------------
4. Java uses the call by value. What is the value that is being passed into routine by the method call in the following ??
double[] rats ={1,2,3};
routine(rats);
correct Answer : A reference to the array object rats.
you may try to deviate with first sentence all by value and may give an answer like "A copy of the array rats" , which would be wrong.
5. Array length
---------------------------------------
int[] myArray = { 1, 2, 3, 4, 5 };
ChangeIt.doIt2(myArray.length);
------------------------------------------
length of the array is taken as property with "lenght" , but with our normal method standards ,we might choose an option like "myArray.length()" - word length with parenthesis.
Remember : length does not have parenthesis.
6. Retrieve values from an array
Most important point in an array is its index starts with "0" - zero. We all know , but some how down the line we miss it. when thing become complicated you will probably my lose this fact.
Output of the below code segment is 12, but you may miss it and select 13, which is wrong- you miss with the index.
So array values starts with 5 and goes with 5,6,7,8, ... until the end.
we retrieve the index "7", that means 8th element , which is value equal to 12.
-----------------------------------------------
int[] num5 = new int[9];
for (int i = 0; i < num5.length; i++) {
num5[i] = i+5;
}
System.out.println(num5[7]);
------------------------------------------------
output
=========================
12
======================
So the highest value associated with the array would be one less than length.
eg : if we define array like
byte[] value = new byte[x];
the highest value for the index would be "x-1"
5. Array length
---------------------------------------
int[] myArray = { 1, 2, 3, 4, 5 };
ChangeIt.doIt2(myArray.length);
------------------------------------------
length of the array is taken as property with "lenght" , but with our normal method standards ,we might choose an option like "myArray.length()" - word length with parenthesis.
Remember : length does not have parenthesis.
6. Retrieve values from an array
Most important point in an array is its index starts with "0" - zero. We all know , but some how down the line we miss it. when thing become complicated you will probably my lose this fact.
Output of the below code segment is 12, but you may miss it and select 13, which is wrong- you miss with the index.
So array values starts with 5 and goes with 5,6,7,8, ... until the end.
we retrieve the index "7", that means 8th element , which is value equal to 12.
-----------------------------------------------
int[] num5 = new int[9];
for (int i = 0; i < num5.length; i++) {
num5[i] = i+5;
}
System.out.println(num5[7]);
------------------------------------------------
output
=========================
12
======================
So the highest value associated with the array would be one less than length.
eg : if we define array like
byte[] value = new byte[x];
the highest value for the index would be "x-1"
Wednesday, April 8, 2015
Relearning the Art of Asking Questions
Relearning the Art of Asking Questions
Proper questioning has become a lost art. The curious four-year-old asks a lot of questions — incessant streams of “Why?” and “Why not?” might sound familiar — but as we grow older, our questioning decreases. In a recent poll of more than 200 of our clients, we found that those with children estimated that 70-80% of their kids’ dialogues with others were comprised of questions. But those same clients said that only 15-25% of their own interactions consisted of questions. Why the drop off?
Think back to your time growing up and in school. Chances are you received the most recognition or reward when you got the correct answers. Later in life, that incentive continues. At work, we often reward those who answer questions, not those who ask them. Questioning conventional wisdom can even lead to being sidelined, isolated, or considered a threat.
Because expectations for decision-making have gone from “get it done soon” to “get it done now” to “it should have been done yesterday,” we tend to jump to conclusions instead of asking more questions. And the unfortunate side effect of not asking enough questions is poor decision-making. That’s why it’s imperative that we slow down and take the time to ask more — and better — questions. At best, we’ll arrive at better conclusions. At worst, we’ll avoid a lot of rework later on.
Aside from not speaking up enough, many professionals don’t think about how different types of questions can lead to different outcomes. You should steer a conversation by asking the right kinds of questions, based on the problem you’re trying to solve. In some cases, you’ll want to expand your view of the problem, rather than keeping it narrowly focused. In others, you may want to challenge basic assumptions or affirm your understanding in order to feel more confident in your conclusions.
Consider these four types of questions — Clarifying, Adjoining, Funneling, and Elevating — each aimed at achieving a different goal:

Clarifying questions help us better understand what has been said. In many conversations, people speak past one another. Asking clarifying questions can help uncover the real intent behind what is said. These help us understand each other better and lead us toward relevant follow-up questions. “Can you tell me more?” and “Why do you say so?” both fall into this category. People often don’t ask these questions, because they tend to make assumptions and complete any missing parts themselves.
Adjoining questions are used to explore related aspects of the problem that are ignored in the conversation. Questions such as, “How would this concept apply in a different context?” or “What are the related uses of this technology?” fall into this category. For example, asking “How would these insights apply in Canada?” during a discussion on customer life-time value in the U.S. can open a useful discussion on behavioral differences between customers in the U.S. and Canada. Our laser-like focus on immediate tasks often inhibits our asking more of these exploratory questions, but taking time to ask them can help us gain a broader understanding of something.
Funneling questions are used to dive deeper. We ask these to understand how an answer was derived, to challenge assumptions, and to understand the root causes of problems. Examples include: “How did you do the analysis?” and “Why did you not include this step?” Funneling can naturally follow the design of an organization and its offerings, such as, “Can we take this analysis of outdoor products and drive it down to a certain brand of lawn furniture?” Most analytical teams – especially those embedded in business operations – do an excellent job of using these questions.
Elevating questions raise broader issues and highlight the bigger picture. They help you zoom out. Being too immersed in an immediate problem makes it harder to see the overall context behind it. So you can ask, “Taking a step back, what are the larger issues?” or “Are we even addressing the right question?” For example, a discussion on issues like margin decline and decreasing customer satisfaction could turn into a broader discussion of corporate strategy with an elevating question: “Instead of talking about these issues separately, what are the larger trends we should be concerned about? How do they all tie together?” These questions take us to a higher playing field where we can better see connections between individual problems.
In today’s “always on” world, there’s a rush to answer. Ubiquitous access to data and volatile business demands are accelerating this sense of urgency. But we must slow down and understand each other better in order to avoid poor decisions and succeed in this environment. Because asking questions requires a certain amount of vulnerability, corporate cultures must shift to promote this behavior. Leaders should encourage people to ask more questions, based on the goals they’re trying to achieve, instead of having them rush to deliver answers. In order to make the right decisions, people need to start asking the questions that really matter.
The Science Of Why You Should Spend Your Money On Experiences, Not Things
The Science Of Why You Should Spend Your Money On Experiences, Not Things
You don't have infinite money. Spend it on stuff that research says makes you happy.
Most people are in the pursuit of happiness. There are economists who think happiness is the best indicator of the health of a society. We know that money can make you happier, though after your basic needs are met, it doesn't make you that much happier. But one of the biggest questions is how to allocate our money, which is (for most of us) a limited resource.
There's a very logical assumption that most people make when spending their money: that because a physical object will last longer, it will make us happier for a longer time than a one-off experience like a concert or vacation. According to recent research, it turns out that assumption is completely wrong.
"One of the enemies of happiness is adaptation," says Dr. Thomas Gilovich, a psychology professor at Cornell University who has been studying the question of money and happiness for over two decades. "We buy things to make us happy, and we succeed. But only for a while. New things are exciting to us at first, but then we adapt to them."

So rather than buying the latest iPhone or a new BMW, Gilovich suggests you'll get more happiness spending money on experiences like going to art exhibits, doing outdoor activities, learning a new skill, or traveling.
Gilovich's findings are the synthesis of psychological studies conducted by him and others into the Easterlin paradox, which found that money buys happiness, but only up to a point. How adaptation affects happiness, for instance, was measured in a study that asked people to self-report their happiness with major material and experiential purchases. Initially, their happiness with those purchases was ranked about the same. But over time, people's satisfaction with the things they bought went down, whereas their satisfaction with experiences they spent money on went up.
It's counterintuitive that something like a physical object that you can keep for a long time doesn't keep you as happy as long as a once-and-done experience does. Ironically, the fact that a material thing is ever present works against it, making it easier to adapt to. It fades into the background and becomes part of the new normal. But while the happiness from material purchases diminishes over time, experiences become an ingrained part of our identity.
"Our experiences are a bigger part of ourselves than our material goods," says Gilovich. "You can really like your material stuff. You can even think that part of your identity is connected to those things, but nonetheless they remain separate from you. In contrast, your experiences really are part of you. We are the sum total of our experiences."
One study conducted by Gilovich even showed that if people have an experience they say negatively impacted their happiness, once they have the chance to talk about it, their assessment of that experience goes up. Gilovich attributes this to the fact that something that might have been stressful or scary in the past can become a funny story to tell at a party or be looked back on as an invaluable character-building experience.
Another reason is that shared experiences connect us more to other people than shared consumption. You're much more likely to feel connected to someone you took a vacation with in Bogotá than someone who also happens to have bought a 4K TV.
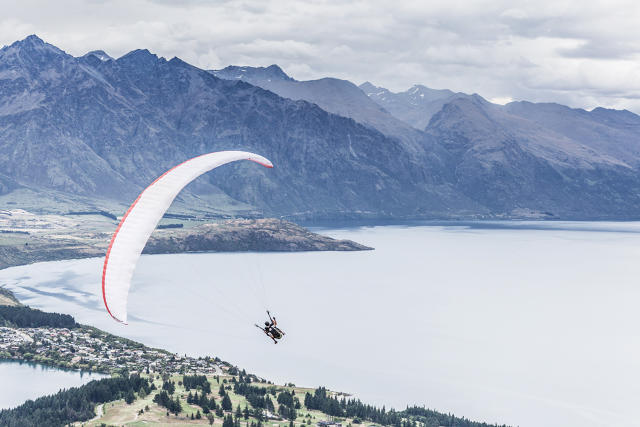
"We consume experiences directly with other people," says Gilovich. "And after they're gone, they're part of the stories that we tell to one another."
And even if someone wasn't with you when you had a particular experience, you're much more likely to bond over both having hiked the Appalachian Trail or seeing the same show than you are over both owning Fitbits.
You're also much less prone to negatively compare your own experiences to someone else's than you would with material purchases. One study conducted by researchers Ryan Howell and Graham Hill found that it's easier to feature-compare material goods (how many carats is your ring? how fast is your laptop's CPU?) than experiences. And since it's easier to compare, people do so.
"The tendency of keeping up with the Joneses tends to be more pronounced for material goods than for experiential purchases," says Gilovich. "It certainly bothers us if we're on a vacation and see people staying in a better hotel or flying first class. But it doesn't produce as much envy as when we're outgunned on material goods."
Gilovich's research has implications for individuals who want to maximize their happiness return on their financial investments, for employers who want to have a happier workforce, and policy-makers who want to have a happy citizenry.
"By shifting the investments that societies make and the policies they pursue, they can steer large populations to the kinds of experiential pursuits that promote greater happiness," write Gilovich and his coauthor, Amit Kumar, in their recent article in the academic journal Experimental Social Psychology.
If society takes their research to heart, it should mean not only a shift in how individuals spend their discretionary income, but also place an emphasis on employers giving paid vacation and governments taking care of recreational spaces.
"As a society, shouldn't we be making experiences easier for people to have?" asks Gilovich.
Subscribe to:
Posts (Atom)